Hello,
I am a android developer and I want to get data of my table(in here: friend’s name and friend’s email) with user where (Like this: userEmail=’my email or user’s email’) but the problem is I am getting repeated data of my table (like: only one person’s name and email is getting) so what is the solution of this please replay.
Thank you.
here is the output in app:
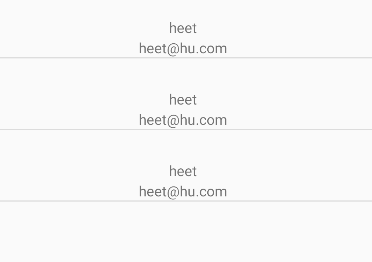
in my table:
Hello,
Could you please share a screenshot of the following:
set a breakpoint where you get data back from the server, and inspect the response you get, take a screenshot and post it here… I would like to see that duplicate data is there and it is not a UI issue.
Regards,
Mark
Here is my MainActivity:
public class MainActivity extends AppCompatActivity {
// parameters:
ListView listView;
static List<Users_friends> list;
Friend_adapter friend_adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
listView=findViewById(R.id.listView);
// object of table:
Users_friends firedns=new Users_friends();
firedns.setF_email("heet@hu.com");
firedns.setF_name("heet");
firedns.setUserEmail("heet@kanabar.com");
DataQueryBuilder dataQueryBuilder=DataQueryBuilder.create();
Log.i("id",Defaults.user.getUserId());
dataQueryBuilder.setWhereClause("ownerId = '"+Defaults.user.getUserId()+"'");
// adding friend:
// dataQueryBuilder.setGroupBy("f_name");
// Backendless.Persistence.of(Users_friends.class).save(firedns, new AsyncCallback<Users_friends>() {
// @Override
// public void handleResponse(Users_friends response) {
// Toast.makeText(MainActivity.this, "created", Toast.LENGTH_SHORT).show();
// }
//
// @Override
// public void handleFault(BackendlessFault fault) {
//
// Toast.makeText(MainActivity.this, "problem1 "+fault.getMessage(), Toast.LENGTH_LONG).show();
// }
// });
// getting data:
Backendless.Persistence.of(Users_friends.class).find(dataQueryBuilder, new AsyncCallback<List<Users_friends>>() {
@Override
public void handleResponse(List<Users_friends> response) {
list=response;
Log.i("f-name",list.get(0).getF_name());
Log.i("f-name",list.get(1).getF_name());
friend_adapter=new Friend_adapter(MainActivity.this,R.layout.friends_list,list);
listView.setAdapter(friend_adapter);
}
@Override
public void handleFault(BackendlessFault fault) {
Log.i("problem2",fault.getMessage());
}
});
}
}
Custom adatapter:
public class Friend_adapter extends ArrayAdapter<Users_friends> {
TextView f_email,f_name;
Context context;
List<Users_friends> list;
int resource;
public Friend_adapter(@NonNull Context context, int resource, @NonNull List<Users_friends> objects) {
super(context, resource, objects);
this.context=context;
list=objects;
this.resource=resource;
}
@SuppressLint("ViewHolder")
@NonNull
@Override
public View getView(int position, @Nullable View convertView, @NonNull ViewGroup parent) {
LayoutInflater inflater=(LayoutInflater) LayoutInflater.from(context);
convertView=inflater.inflate(resource,parent,false);
f_email=convertView.findViewById(R.id.f_email);
f_name=convertView.findViewById(R.id.f_name);
//setting data to the UI given by response:
f_name.setText(getItem(position).getF_name());
f_email.setText(getItem(position).getF_email());
return convertView;
}
}
Table object:
public class Users_friends {
static String f_name;
static String f_email;
static String userEmail;
public Users_friends() {
f_email=f_name=userEmail=null;
}
public String getUserEmail() {
return userEmail;
}
public void setUserEmail(String userEmail) {
Users_friends.userEmail = userEmail;
}
public String getF_name() {
return f_name;
}
public void setF_name(String f_name) {
this.f_name = f_name;
}
public String getF_email() {
return f_email;
}
public void setF_email(String f_email) {
this.f_email = f_email;
}
}
app screenshot:
Table screenshot:
Did you set a breakpoint to check in the debugger what the server returns?
@mark-piller
Yes I did and it and it’s returning same output
Could you please attach a screenshot showing the value of the “response” argument in a debugger?
I am asking to see the value of the “response” argument. Not a logcat output, but a value from an inspector in debugger.
Here’s information on how to debug Android apps, set breakpoints and inspect variables:
Regards,
Mark
The problem is actually in your code, there is nothing wrong with Backendless.
The fields which represent data for individual objects are declared “static”. As a result, as soon as you assign a value, it will be updated for all objects.
2 Likes
@mark-piller
Thank you so much sir. It really helped me. Thank you again.