I am trying to save a relationship to my currentUser, when I do it, I get the above error. Does anyone know what the problem could be?
func uploadAddedRetailers(checkedRetailers:[RetailerPage]) {
let user = backendless.userService.currentUser
user.setProperty("followedRetailers", object: checkedRetailers)
backendless.data.of(BackendlessUser.ofClass()).save(user, response: { (response) in
print("response is \(response)")
}) { (fault) in
print("fault is \(fault)")
}
}
Please provide here your appId and RetailerPage class.
App Id:
3B865B6E-3046-D761-FF41-7D1C8DC34400
Retailer Page: Attached
Thank you!
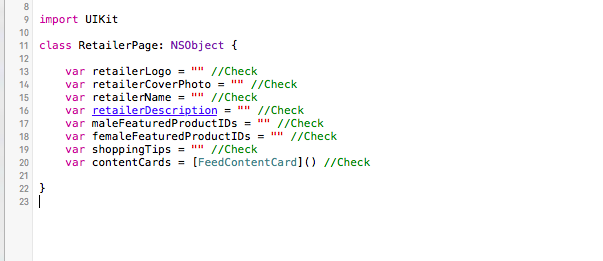
Rich, please provide your class as text, I need to copy/paste it
class RetailerPage: NSObject {
var retailerLogo = "" //Check
var retailerCoverPhoto = "" //Check
var retailerName = "" //Check
var retailerDescription = "" //Check
var maleFeaturedProductIDs = "" //Check
var femaleFeaturedProductIDs = "" //Check
var shoppingTips = "" //Check
var contentCards = [FeedContentCard]() //Check
}
Here is the table schema in Backendless as well. The user table has a one to many relationship with this attached table.
export_2016_05_17_14_43_28.zip (319B)
And FeedContentCard as text please
class FeedContentCard: NSObject {
var featuredProductId = ""
var gender = ""
var offerDescription = ""
var offerTitle = ""
var photos = ""
var retailerLogo : UIImage!
var retailerCoverPhoto :UIImage!
var retailerName = ""
var shoppingTip = ""
var video = ""
var retailer: RetailerPage!
}
You cannot save UIImage object as Data table field - only as NSData
class FeedContentCard: NSObject {
var featuredProductId = ""
var gender = ""
var offerDescription = ""
var offerTitle = ""
var photos = ""
var retailerLogo = ""
var retailerCoverPhoto = ""
var retailerName = ""
var shoppingTip = ""
var video = ""
var retailer: RetailerPage!
}
-Here is the new FeedContent Card and I still get the same error.
fault is FAULT = ‘2004’ [Property name contains invalid characters: 1038728E-5F1D-93FF-FF03-86D590A75C00] <Property name contains invalid characters: 1038728E-5F1D-93FF-FF03-86D590A75C00>
We are also this error when opening backendless feedbcontentcard table. This is in the backendless console itself.
Now your FeedContentCard & RetailerPage tables are broken. Could you remove them and fit again?
We just recreated them. They are working now. But the same original issues is still there with us getting the following error: “Property name contains invalid characters”
I just created a sample func, it fetches the all existing RetailerPage and sets them as ‘followedRetailers’ user property:
func updateUserRetailerPage() {
//
loginUser("bob@backendless.com", password: "greeng0blin")
let user = backendless.userService.currentUser
print("CURRENT USER: \(user)")
//
backendless.persistenceService.of(RetailerPage.ofClass()).find(
BackendlessDataQuery(),
response: { (results : BackendlessCollection!) -> () in
let currentPage = results.getCurrentPage() as! [RetailerPage]
print("Loaded \(currentPage.count) RetailerPage objects")
self.uploadAddedRetailers(currentPage) // it is your func above
},
error: { ( fault : Fault!) -> () in
print("Server reported an error: \(fault)")
}
)
}
It works, you can see my record on your app dashboard. Please try this func with your login/password (which throws a fault) - and let me know how it goes.
We are trying to add a relationship between a user and a retailer. In this scenario, we are adding retailers that a user follows the same way a user would follow a friend on Instagram. How do we append that relationship in the user table. We can do it in any table other than the user table. When we use the logic above (posted by Rich) to do it for the user table, it gives us an error. In the docs there is an append command used for other tables as follows: phoneBook.contacts.append(contactToAdd). How do we do this for the user table?
Unfortunately, this did not work for us to solve the issue we are having. Thank you so much for the fast response today.
Hi Nevin,
Here is a sample (in obj-c, but I think it doesn’t matter):
-(void)testUpdateUserWithRelatedUsers {
@try {
[self loginUser:@"spiday@backendless.com" password:@"greeng0blin"];
BackendlessUser *currentUser = backendless.userService.currentUser;
// create 1:N relation 'friends'
NSMutableArray *friends = [NSMutableArray new];
// add a last user to the friends
BackendlessUser *user = [[backendless.persistenceService of:BackendlessUser.class] findLast];
NSLog(@"Last User has been found: %@", user);
if (!user) {
return;
}
[friends addObject:user];
[currentUser setProperty:@"friends" object:friends];
[backendless.userService update:currentUser];
NSLog(@"Current User has been updated (1): %@", currentUser);
// remove a last user from the friends
friends = [currentUser getProperty:@"friends"];
NSLog(@"FRIENDS: %@", friends);
for (BackendlessUser *friend in friends) {
if ([friend.objectId isEqualToString:user.objectId]) {
[friends removeObject:friend];
break;
}
}
[currentUser setProperty:@"friends" object:friends];
[backendless.userService update:currentUser];
NSLog(@"Current User has been updated (2): %@", currentUser);
// add a first user to the friends
user = [[backendless.persistenceService of:BackendlessUser.class] findFirst];
NSLog(@"First User has been found: %@", user);
if (!user) {
return;
}
friends = [currentUser getProperty:@"friends"];
[friends addObject:user];
[currentUser setProperty:@"friends" object:friends];
[backendless.userService update:currentUser];
NSLog(@"Current User has been updated (3): %@", currentUser);
}
Regards,
Slava
Do you have the code in swift? Sorry I am much more familiar in swift.
Here is my code for my situation in Swift. How does that look?
class func testAddRetailer() {
let user = backendless.userService.currentUser
let relation = backendless.persistenceService.of(RetailerPage.ofClass()).findFirst() as? RetailerPage
if((relation) != nil) {
var likedPages = [RetailerPage]()
likedPages.append(relation!)
user.setProperty("followedRetailers", object: likedPages)
backendless.userService.update(user, response: { (user) in
print("user updated")
}, error: { (fault) in
print("fault is \(fault)")
})
}
else {
}
}
See this comment in another thread.