Hello,
I have the ‘kind_of_products’ table with two fields, ‘new’ and ‘used’.
How can I make when creating a new product I set the ‘kind_of_products_id’ equal to the ID of ‘new’ or ‘used’? I can do that by the browser but not with the javascript.
Thanks.
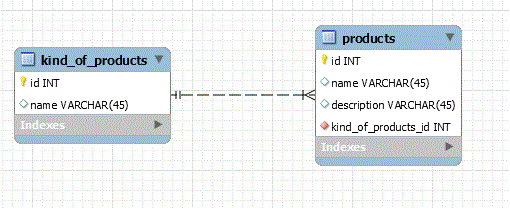
Hi, Raul!
I assume that you have table “Product”, and your task is to reference a “kind_of_product” object by “Product” object. In order to do that a relation should be established. It can be done through the console. Define a column “related_kind_of_product” with type “Data object relationship” to table “kind_of _product”.
More information and samples about working with relations from JS you can find here: http://backendless.com/documentation/data/js/data_relations_save_update.htm
best regards,
Alex Navara
Hello Alex,
Thank you for your support.
Yeah, I know how to create this kind of relationship, but my problem is when the object already exists.
Using this way the code will create a new kind_of_product and I want to use an existing object.
For example: using MySQL I can set the kind_of_product ID in the products table. How can I set this ID in the products table (without create a new one).
In such case you should retrieve the existing object which you want to make related (in case you haven’t yet) and set it as a property to your newly created object. After that you save the newly created object and now have a relationship with the existing one.
Hello, Paul!
To do it, you should retrieve the object you want to establish relation with. Here I’ve made a small example for you. It creates new Product object which references first object of “kind_of_product” class.
var APP_ID = "",
SECRET_KEY = "",
VERSION = "";
function initApp() {
Backendless.initApp( APP_ID, SECRET_KEY, VERSION );
}
function kind_of_product( args )
{
args = args || {};
this.name = args["name"] || "";
this.used = args["used"] || "";
}
function Product( args )
{
args = args || {};
this.name = args["name"] || "";
this.kind = args["kind"] || "";
}
function saveObject() {
var kind = new kind_of_product({
name : "new",
used : false
});
Backendless.Persistence.of( kind_of_product ).save( kind );
}
function establishRelation() {
var kind = Backendless.Persistence.of( kind_of_product ).findFirst();
var bicycle = new Product({
name : "bicycle",
kind : kind
});
Backendless.Persistence.of( Product ).save( bicycle );
}
initApp();
saveObject();
establishRelation();
In order to retrieve already existing object using parameters Backendless.DataQuery class should be used. Here you have an example:
var dataQuery = new Backendless.DataQuery();
dataQuery.condition = "objectId='your_object_id'";
Backendless.Persistence.of(YourClass).find(dataQuery);
More about advanced searching you can read here: http://backendless.com/documentation/data/js/data_search_and_query.htm
About saving objects with relations: https://backendless.com/feature-1-saving-objects-with-relations/
if you have more questions, or need help - please do not hesitate to contact us.
Alex Navara
Thank you, it worked 
I have another question. How do I search for a ‘kind’ in Product? Example:
select * from Products where Products.kind = ‘some_ID_of_kind_of_products’
You can use the same request, but change the “where clause”. Here is an example:
var dataQuery = new Backendless.DataQuery();
dataQuery.condition = "kind='kind_of_product'";
Backendless.Persistence.of(Products).find(dataQuery);
You can use partial match searching with “where clause” with “LIKE”:
var dataQuery = new Backendless.DataQuery();
dataQuery.condition = "kind LIKE '%part_of_kind%'";
Backendless.Persistence.of(YourClass).find(dataQuery);
best regards,
Alex Navara
var dataQuery = new Backendless.DataQuery();
dataQuery.condition = "kind_of_conditions='5F54E0FE-58DD-390F-FF6A-89BCBF52F800'";
var ads = Backendless.Persistence.of( new $rootScope.Classes.Ads() ).find(dataQuery);
Result console:
Object { message: “Invalid where clause: Unknown column ‘where clause’”, code: 1017, statusCode: 400 }
REST Console browser also gives the same error. The kind_of_conditions column is a relationship with another table.
Try this:
dataQuery.condition = "kind_of_conditions.objectId='5F54E0FE-58DD-390F-FF6A-89BCBF52F800'";
It worked, thank you for your support.